There was a time when we used to discuss document content and feedback in long email threads with multiple attachments and different file versions. Now we can simply use annotations to markup the document with messages and replies and send it. In this article, you will learn how to programmatically annotate PDF documents in Java with your application. Additionally, we will see how to remove annotations from PDF files using the same Java API.
The following are the topics discussed briefly below:
- Java API to Work with Annotations in PDF
- Add Annotations to PDF in Java
- Remove Annotations from PDF in Java
PDF Annotator Java API
To deal with annotations of your document and images within your Java applications, GroupDocs provides GroupDocs.Annotation for Java. Using the API, you can add, remove, and extract annotations from word-processing documents, spreadsheets, presentations, images, email messages, Visio drawings, some AutoCAD, and digital imaging formats like DICOM. Furthermore, the API allows annotating PDF files. You may have a look at the documentation to know about the long list of supported document formats for annotation.
Download and Configure
Get the annotation library from downloads or just add the following pom.xml configuration in your Maven-based Java applications to try the examples of this article as well the many more example available on GitHub. For the details, you may visit the API Reference.
<repository>
<id>GroupDocsJavaAPI</id>
<name>GroupDocs Java API</name>
<url>http://repository.groupdocs.com/repo/</url>
</repository>
<dependency>
<groupId>com.groupdocs</groupId>
<artifactId>groupdocs-annotation</artifactId>
<version>20.2</version>
</dependency>
Add Annotations to PDF in Java
Let’s quickly jump to add some of the different kinds of annotations to the PDF document. As there are many different types of annotation, we may not cover all in this article. I will just mention them, and you may learn about each annotation individually.
-
Area / Rectangle annotation
-
Arrow
-
Distance
-
Ellipse
-
Highlight
-
Link
-
Point
-
Polyline
-
Replacement
-
Resource Redaction
-
Strikeout
-
Text Field
-
Text Redaction
-
Underline
-
Watermark
Let’s start adding some of these in a PDF document.
Add Arrow Annotation to PDF using Java
The following are the steps to add arrow annotation to a PDF document.

- Load the PDF document using the Annotator class.
- Initialize arrow annotation using ArrowAnnotation class.
- Set the position and size of the arrow using setBox method of ArrowAnnotation.
- Add the created arrow annotation to the Annotator object.
- Save the annotated PDF by providing the path using the save method.
The following code sample shows how to add arrow annotation to a PDF document using Java.
Insert Rectangle or Area Annotation to PDF using Java
You can customize any annotation while adding it to the document. The following are the steps to add rectangle or area annotation to a PDF document with little more customizations. It is similar to adding Arrow annotation but uses AreaAnnotation class in place of ArrowAnnotation.
- Load the PDF document using the Annotator class.
- Initialize rectangle annotation using AreaAnnotation class.
- Set the position and size of the rectangle using setBox method of AreaAnnotation.
- Set other properties like color, background, opacity, style, pen width, or even messages, and time.
- Add the created rectangle annotation to the Annotator object.
- Save the annotated PDF by providing the path using the save method.

The following code sample shows how to add rectangle/area annotation to a PDF document using Java.
Add Oval or Ellipse Annotation to PDF using Java
The following are the steps to add oval annotation or ellipse annotation to a PDF document.
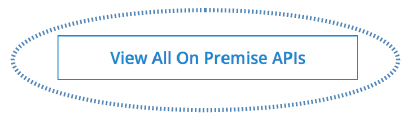
- Load the PDF document using the Annotator class.
- Initialize ellipse annotation using EllipseAnnotation class.
- Set the position and size of the ellipse using setBox method of EllipseAnnotation.
- Add the created ellipse annotation to the Annotator object.
- Save the annotated PDF by providing the path using the save method.
The following code sample shows how to add oval or ellipse annotation to a PDF document using Java.
Insert Distance Annotation to PDF using Java
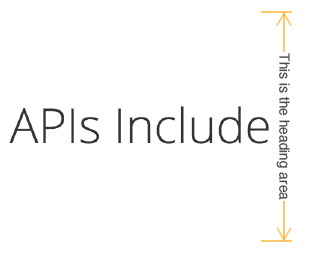
You can also add the distance annotation to show the distance between two points. The following are the steps to add distance annotation to the PDF document.
- Load the PDF document using the Annotator class.
- Initialize distance annotation using DistanceAnnotation class.
- Set the size and position of the annotation using setBox method of DistanceAnnotation.
- Add the created distance annotation to the Annotator object.
- Save the annotated PDF by providing the path using the save method.
The following code sample shows how to add distance annotation to a PDF document using Java.
Complete Code
To sum up, here is the Java code with the output showing all the added annotations and messages with replies using the mentioned Java code.

The following code below adds, arrow, rectangle, ellipse, distance annotations, messages, and replies to a PDF file.
Remove Annotations from PDF in Java
The following steps show how to remove all the annotations from PDF files in Java.
- Load the PDF document using the Annotator class.
- Initialize saving Options using SaveOptions class.
- Set the annotation types to None.
- Save the PDF file having all the annotations removed, by providing the path using the save method.
The following Java code removes annotations from a PDF file.
Conclusion
In short, you have learned how to add annotations to PDF within Java applications. Further, you have seen how to remove all the annotations from any PDF file. Now, you should be confident to build your own document annotator Java application. It can support different types of annotations using GroupDocs.Annotation for Java.
For more details, options, and examples, you can visit the documentation and the GitHub repository. For further queries, contact the support on the forum.