JSON is a formatted and readable data interchange format to transmit data with attributes. However, the large data in JSON format is not very presentable and easily understandable. We mostly need to convert the large JSON data into a presentable format. This article will guide you to convert JSON data into PDF and MS Word reports in Java using a simple template.
Report Generation Java API
I will be using GroupDocs.Assembly for Java API to generate reports from the provided JSON data and template in DOCX and TXT format. It also supports automatic report generation in multiple formats from CSV, XML data sources.
Download or Configure
You may download the JAR file from the downloads section, or just get the repository and dependency configurations for the pom.xml of your maven-based Java applications.
<repository>
<id>GroupDocsJavaAPI</id>
<name>GroupDocs Java API</name>
<url>http://repository.groupdocs.com/repo/</url>
</repository>
<dependency>
<groupId>com.groupdocs</groupId>
<artifactId>groupdocs-assembly</artifactId>
<version>21.1</version>
</dependency>
Generate PDF Report from JSON Data in Java
Let’s quickly jumps to the steps that will lead you to convert JSON data into the formatted PDF report.
- Get JSON data source
- Define template according to JSON data
- Provide JSON data source and template to simple java code for report generation.
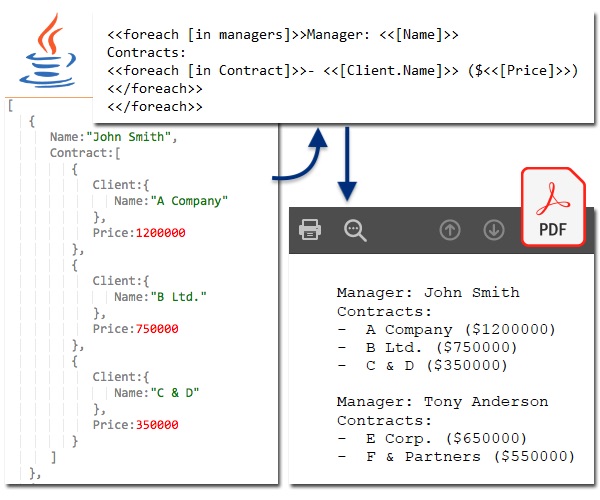
JSON Data
For the PDF report generation, I will be using the following sample JSON data of managers and their respective clients and details.
\[
{
"Name":"John Smith","Contract":\[
{"Client":{"Name":"A Company"},"Price":1200000},
{"Client":{"Name":"B Ltd."},"Price":750000},
{"Client":{"Name":"C & D"},"Price":350000}\]
},
{
"Name":"Tony Anderson","Contract":\[
{"Client":{"Name":"E Corp."},"Price":650000},
{"Client":{"Name":"F & Partners"},"Price":550000}\]
},
{
"Name":"July James","Contract":\[
{"Client":{"Name":"G & Co."},"Price":350000},
{"Client":{"Name":"H Group"},"Price":250000},
{"Client":{"Name":"I & Sons"},"Price":100000},
{"Client":{"Name":"J Ent."},"Price":100000}\]
}
\]
Template
Define the following template in TXT or DOCX format. This will allow to iterate over Managers and their respective Clients and their details. After that, you can jump to code for report generation.
<<foreach [in managers]>>Manager: <<[Name]>>
Contracts:
<<foreach [in Contract]>>- <<[Client.Name]>> ($<<[Price]>>)
<</foreach>>
<</foreach>>
Java Steps to Generate PDF Report from JSON
Following steps and Java code allows automatic conversion of JSON data into PDF report as per defined template.
- Define JSON data file, .txt template file, and PDF output report file paths.
- Instantiate JsonDataSoure with JSON data file.
- Create DataSourceInfo with defined JsonDataSource.
- Call the assembleDocument method of the DocumentAssembler class to generate the PDF report from the provided JSON data and defined template.
Generate MS Word Report from JSON data in Java
Similarly, like the above PDF report generation, you can easily create the DOCX report by:
- Defining the same template in DOCX format.
- Set the output report document format as DOCX.
- The rest of the code will remain the same to generate MS Word DOCX report from the JSON data.
For more details, options, and examples, you can go through the documentation and GitHub repository. In case of further queries and ambiguities, contact the free support on the forum.
Conclusion
I hope you will feel comfortable building your own Java-based application to generate reports by converting JSON data to PDF format. Similarly, you can generate reports in other formats like DOCX using other data sources like CSV and XML.